In Java, the `LinkedHashSet` is a powerful collection class that combines the best of both worlds: it maintains the insertion order of elements while ensuring uniqueness, just like a `HashSet`. But when should you opt for `LinkedHashSet` over other Set implementations?
Key Features of `LinkedHashSet`:
1. Ordered Elements: Unlike `HashSet`, `LinkedHashSet` maintains the order of elements as they were inserted. This is crucial when the order of processing or output needs to be predictable.
2. Uniqueness: Similar to `HashSet`, `LinkedHashSet` does not allow duplicate elements. If an element already exists in the set, any attempt to add it again will be ignored.
3. Performance: While `LinkedHashSet` is slightly slower than `HashSet` due to the overhead of maintaining the order, it still provides constant time performance (O(1)) for basic operations like add, remove, and contains.
When to Use `LinkedHashSet`?
– Maintaining Order: Use `LinkedHashSet` when you need a collection that maintains the insertion order, such as preserving the sequence of user actions in an application.
– Ensuring Uniqueness: It’s ideal when you need both order and uniqueness, like when storing a list of unique, ordered configuration keys.
Conclusion
`LinkedHashSet` is an excellent choice when you need a collection that guarantees both the uniqueness of elements and the order in which they were added. Its ability to balance these two requirements makes it particularly useful in many real-world applications.
Have you used `LinkedHashSet` in your projects? Share your thoughts or questions below!
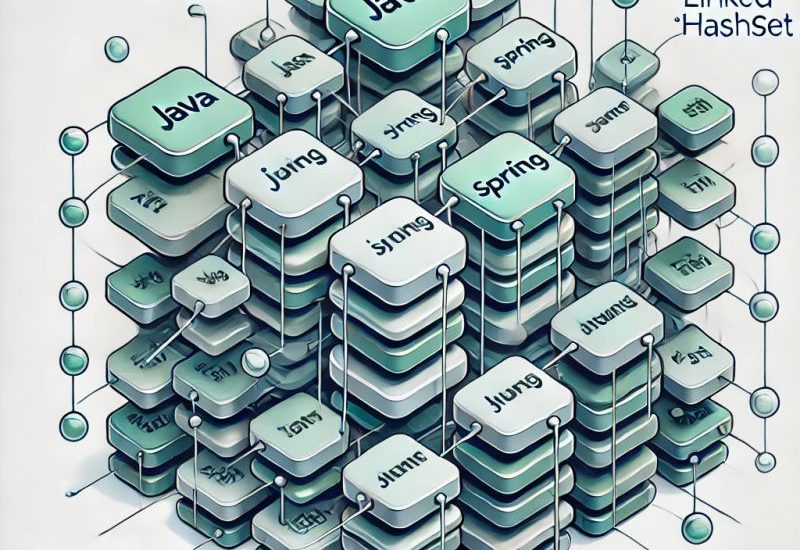
Mastering `LinkedHashSet` in Java: Order with Uniqueness
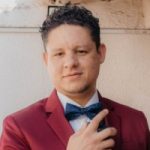