Writing clean code is more than just a best practice—it’s a philosophy that elevates your work from functioning code to maintainable, scalable, and efficient software. Here’s how to embrace clean code principles in Java:
1. Meaningful Names
Choose clear, descriptive names for your variables, methods, and classes. Avoid abbreviations and ensure that each name conveys the purpose of the entity.
2. Keep It Simple (KISS)
Simplicity is the ultimate sophistication. Avoid overcomplicating your code. Focus on writing straightforward solutions that are easy to understand and maintain.
3. DRY (Don’t Repeat Yourself)
Repetition is the enemy of clean code. Consolidate duplicate code by creating reusable methods or classes. This reduces the chances of errors and makes future changes easier to manage.
4. Small Functions
Break down complex tasks into smaller, manageable functions. Each function should do one thing and do it well. This not only enhances readability but also makes debugging and testing more straightforward.
5. Comments Are a Last Resort
While comments can be helpful, they often indicate that your code isn’t as clear as it could be. Aim to write self-explanatory code where comments are rarely needed.
6. Consistent Formatting
Maintain a consistent coding style across your project. Whether it’s indentation, brace placement, or line spacing, consistency in formatting makes your code more readable and easier to navigate.
7. Embrace SOLID Principles
Adopt the SOLID principles (Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, Dependency Inversion) to design robust, flexible, and scalable systems.
By following these clean code practices, you’ll not only create better software but also enhance collaboration and make life easier for anyone who works on your code in the future. Remember, code is written once but read many times—make it a pleasant experience!
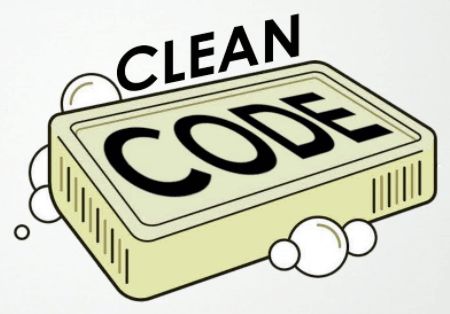
The Art of Clean Code in Java
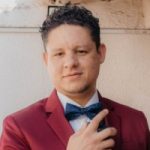